The Learn Python & Django Developer Bundle
What's Included
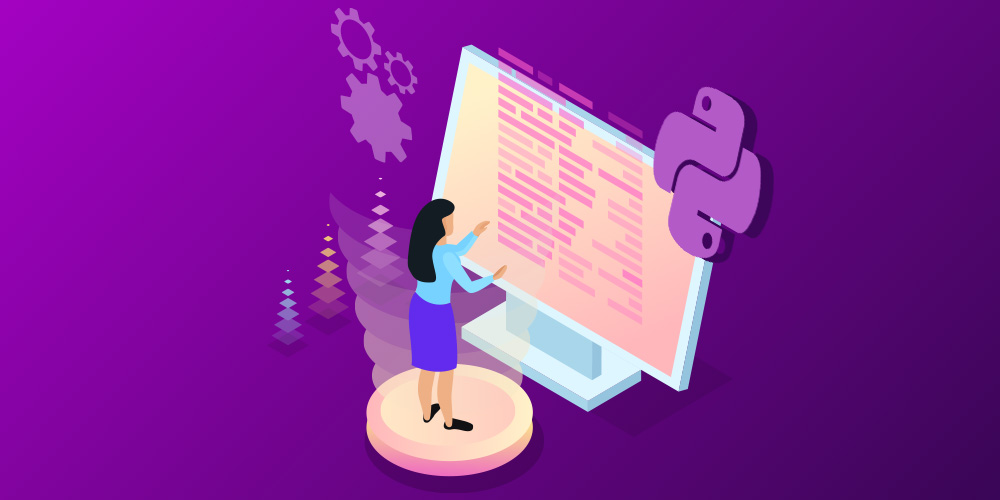
Complete Python Master Class: Learn Python Programming by Building Projects
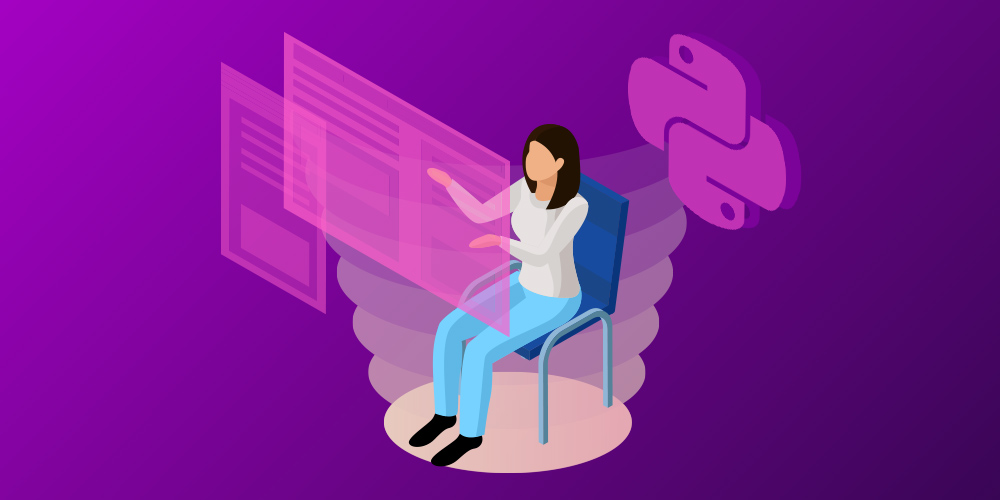
Python Programming Advanced: Understanding Weird Concepts
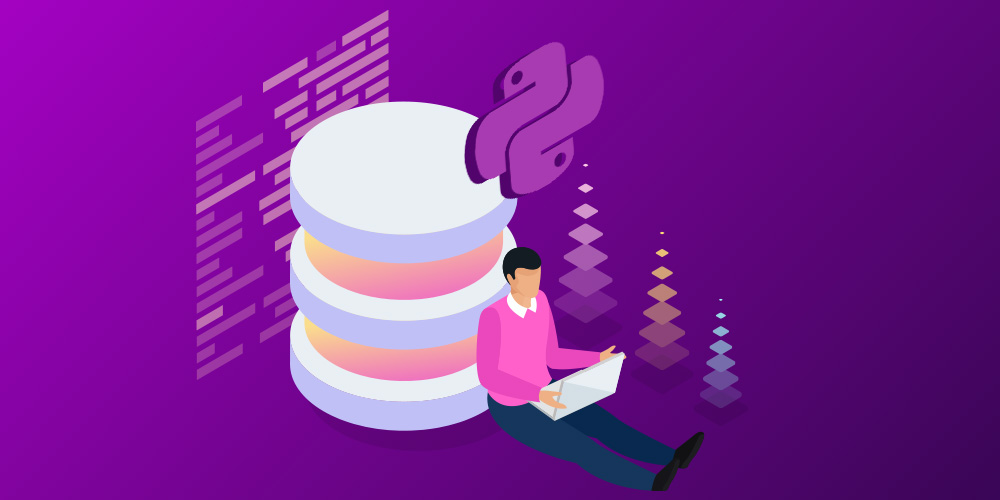
Python GUI Programming: Building Desktop Application with TKinter & SQLite
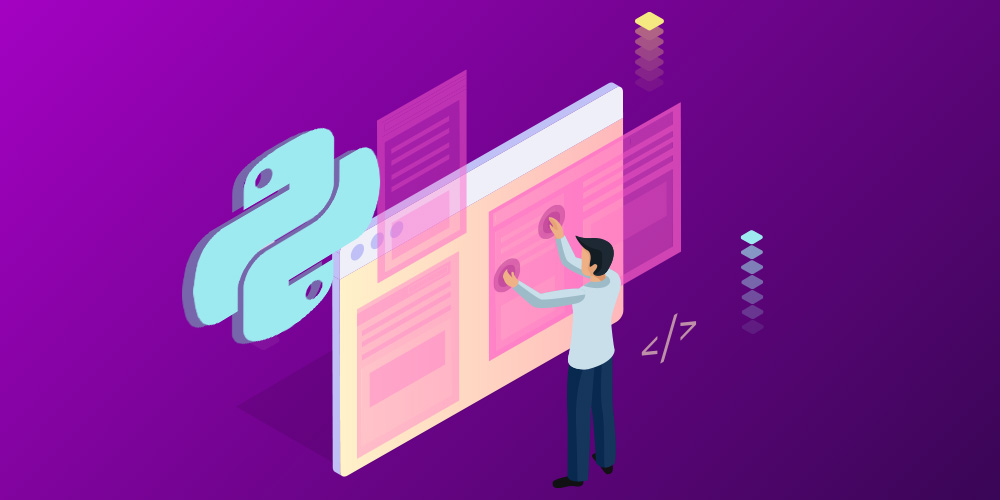
Python Automation Project: Building Web Scraping Bot with Beautiful Soup
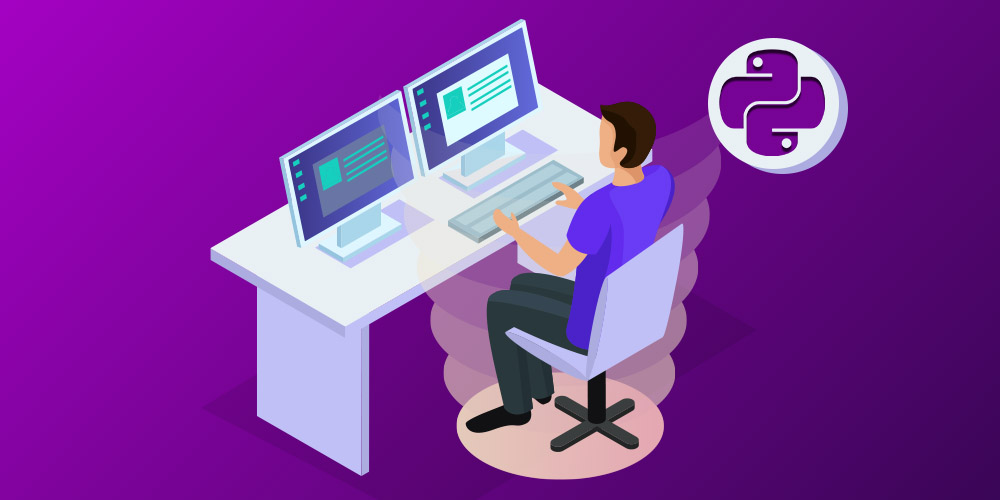
Django Master Class: Complete Web Development with Python
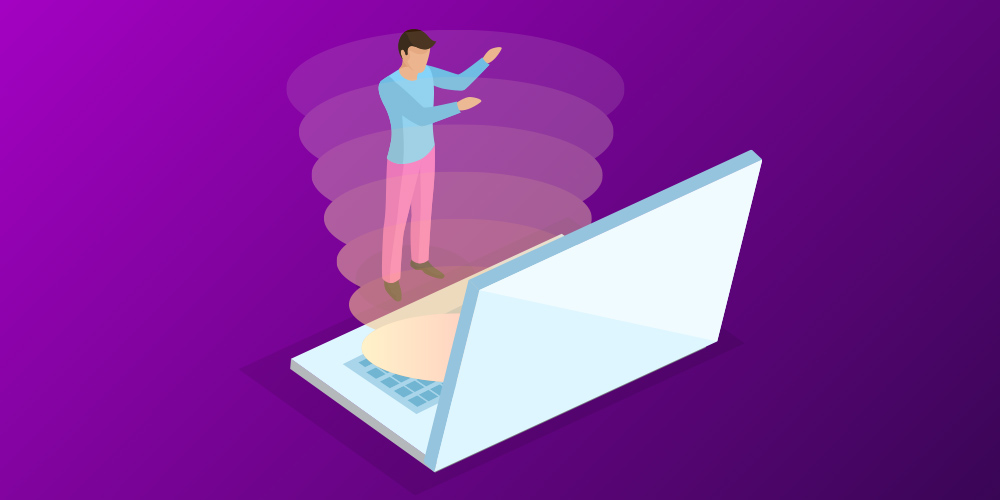
Complete Git & GitHub for Beginners: Practical Bootcamp
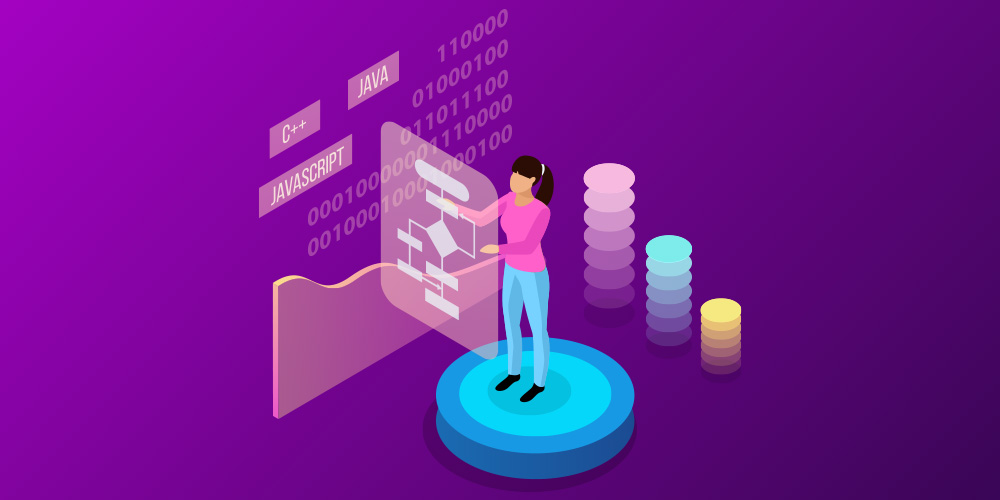
Data Structures & Algorithms: The Complete Master Class
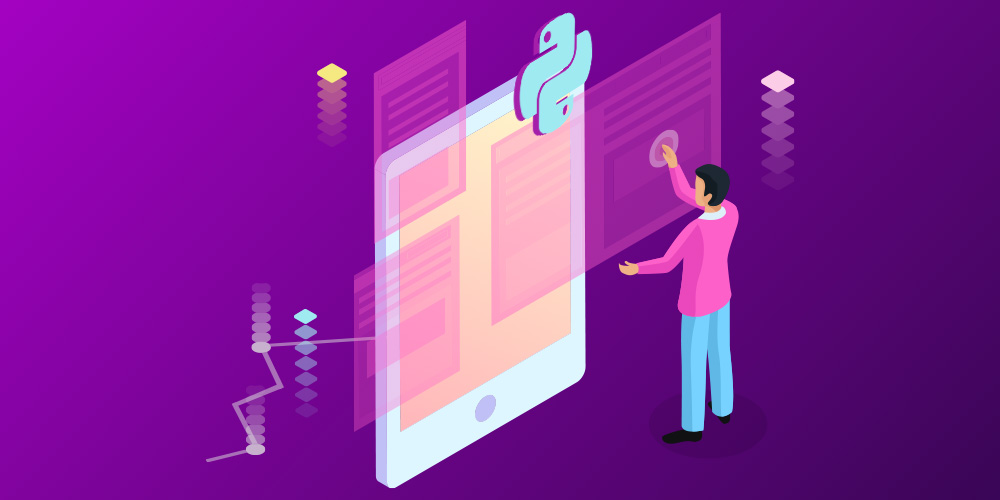
Python Project: Automate Instagram Post Design
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.
Amitabha Ghosh
Though bought on Sep 28, took my first look only today (Oct 12) as I'm now out of country on some urgent work. Expect to be regular from December. Hence my observation and rating are based on only 1% of the course (INTRODUCTION)! My views from this short association are as follow: 1. The presentation was quite nice and clean 2. As I'm a senior citizen, I'd like some way to slow down the video when needed 3. If some parts used Python, a mention would be encouraging to know what Python can do!
Shon Garrison
Very comprehensive! The only downside, it's not organized very well. Hard to tell where to start and where it will end. I ended up on an advanced topic I was not prepared for and had to backtrack. An outline and a plotted course through the material would be good.